Make patches from labels#
Make a set of augmented patches from labels.
The difference between labels and patches is:
Labels are a set of images and annotated ground truths, generated manually, or automatically. Labels can be different sizes.
Patches are a set of images and annotated ground truths generated from labels. They are usually cropped from labels to be all the same size. They are often augmented. 1 label can be used to generate many patches.
from skimage.io import imread
from matplotlib import pyplot as plt
from tnia.deeplearning.dl_helper import quantile_normalization, get_label_paths
from tnia.plotting.plt_helper import imshow_multi2d
import numpy as np
import json
from glob import glob
import pandas as pd
import os
from pathlib import Path
from tnia.deeplearning.augmentation import uber_augmenter
data_path = r'../../data'
parent_path = os.path.join(data_path, 'ladybugs_sparse')
label_path = os.path.join(parent_path, 'labels')
# open the info file
json_name = os.path.join(label_path, 'info.json')
json_ = json.load(open(json_name))
# get number of inputs and number of ground truths for this problem
num_inputs = json_['num_inputs']
num_ground_truths = json_['num_truths']
print('num inputs: ', num_inputs)
print('num ground truth: ', num_ground_truths)
image_label_paths, ground_truths_label_paths = get_label_paths(1, num_ground_truths, label_path)
print('image label paths',image_label_paths)
print("ground_truth_label_paths", ground_truths_label_paths[0])
# get list of tif files in image_label_path
tif_files = glob(str(os.path.join(image_label_paths[0], '*.tif')))
json_files = glob(str(os.path.join(image_label_paths[0], '*.json')))
print()
for tif_file in tif_files:
print("tif_file: ", tif_file)
patch_path= os.path.join(parent_path, 'patches')
if not os.path.exists(patch_path):
os.mkdir(patch_path)
axes = 'YXC'
sub_sample = 1
num inputs: 1
num ground truth: 1
image label paths [PosixPath('/home/bnorthan/code/i2k/tnia/notebooks-and-napari-widgets-for-dl/data/ladybugs_sparse/labels/input0')]
ground_truth_label_paths /home/bnorthan/code/i2k/tnia/notebooks-and-napari-widgets-for-dl/data/ladybugs_sparse/labels/ground truth0
tif_file: /home/bnorthan/code/i2k/tnia/notebooks-and-napari-widgets-for-dl/data/ladybugs_sparse/labels/input0/6410573_8085113_0.tif
tif_file: /home/bnorthan/code/i2k/tnia/notebooks-and-napari-widgets-for-dl/data/ladybugs_sparse/labels/input0/8220954_10897041_0.tif
Augment#
In this step we loop through all the labels, normalize them and augment each label to create patches.
The code is almost identical to what was done in the 20_create_patches
notebook from the previous section. The one important difference is that we subtract 1 from the image so that all ‘unlabeled’ pixels are negative, and background pixels are 0.
patch_size = 256
number_patches = 200
# create a df to save the label names in
df = pd.DataFrame(columns=['base_name', 'xstart', 'ystart', 'xend', 'yend'])
for i in range(len(tif_files)):
# User input set index of label
# get tif name
tif_name = os.path.basename(tif_files[i])
print('tiff name is', tif_name)
image = imread(str(image_label_paths[0]/ (tif_name)))
image = image
# read labels (there can be more than one class)
labels = []
for ground_truths_label_path in ground_truths_label_paths:
label = imread(os.path.join(ground_truths_label_path / (tif_name)))
label = label-1
labels.append(label)
# show labels image
images_to_show=[]
titles = []
images_to_show.append(image)
titles.append("image")
for label in labels:
images_to_show.append(label)
titles.append("mask")
fig = imshow_multi2d(images_to_show, titles, 1, len(images_to_show), width=20, height=10)
image = quantile_normalization(image, quantile_low = .01, quantile_high=.998, clip = False).astype(np.float32)
uber_augmenter(image, labels, Path(patch_path), 'grid', patch_size, number_patches, do_random_brightness_contrast = False, do_random_gamma=False)
uber_augmenter(image, labels, Path(patch_path), 'grid', patch_size, number_patches, do_random_brightness_contrast = True, do_random_gamma=False)
uber_augmenter(image, labels, Path(patch_path), 'grid', patch_size, number_patches, do_random_brightness_contrast = True, do_random_gamma=True)
uber_augmenter(image, labels, Path(patch_path), 'grid', patch_size, number_patches, do_random_brightness_contrast = True, do_random_gamma=True, do_color_jitter = True)
tiff name is 6410573_8085113_0.tif
/home/bnorthan/code/i2k/tnia/tnia-python/tnia/deeplearning/augmentation.py:227: UserWarning: /home/bnorthan/code/i2k/tnia/notebooks-and-napari-widgets-for-dl/data/ladybugs_sparse/patches/ground truth0/grid_31.tif is a low contrast image
imsave(label_name, label_aug[j])
/home/bnorthan/code/i2k/tnia/tnia-python/tnia/deeplearning/augmentation.py:227: UserWarning: /home/bnorthan/code/i2k/tnia/notebooks-and-napari-widgets-for-dl/data/ladybugs_sparse/patches/ground truth0/grid_353.tif is a low contrast image
imsave(label_name, label_aug[j])
/home/bnorthan/mambaforge/envs/easy_augment_pytorch/lib/python3.12/site-packages/albumentations/augmentations/functional.py:747: RuntimeWarning: invalid value encountered in power
return np.power(img, gamma)
/home/bnorthan/code/i2k/tnia/tnia-python/tnia/deeplearning/augmentation.py:227: UserWarning: /home/bnorthan/code/i2k/tnia/notebooks-and-napari-widgets-for-dl/data/ladybugs_sparse/patches/ground truth0/grid_459.tif is a low contrast image
imsave(label_name, label_aug[j])
/home/bnorthan/mambaforge/envs/easy_augment_pytorch/lib/python3.12/site-packages/pydantic/main.py:364: UserWarning: Pydantic serializer warnings:
Expected `Union[float, tuple[float, float]]` but got `list` - serialized value may not be as expected
Expected `Union[float, tuple[float, float]]` but got `list` - serialized value may not be as expected
Expected `Union[float, tuple[float, float]]` but got `list` - serialized value may not be as expected
return self.__pydantic_serializer__.to_python(
/home/bnorthan/code/i2k/tnia/tnia-python/tnia/deeplearning/augmentation.py:227: UserWarning: /home/bnorthan/code/i2k/tnia/notebooks-and-napari-widgets-for-dl/data/ladybugs_sparse/patches/ground truth0/grid_597.tif is a low contrast image
imsave(label_name, label_aug[j])
/home/bnorthan/code/i2k/tnia/tnia-python/tnia/deeplearning/augmentation.py:227: UserWarning: /home/bnorthan/code/i2k/tnia/notebooks-and-napari-widgets-for-dl/data/ladybugs_sparse/patches/ground truth0/grid_647.tif is a low contrast image
imsave(label_name, label_aug[j])
tiff name is 8220954_10897041_0.tif
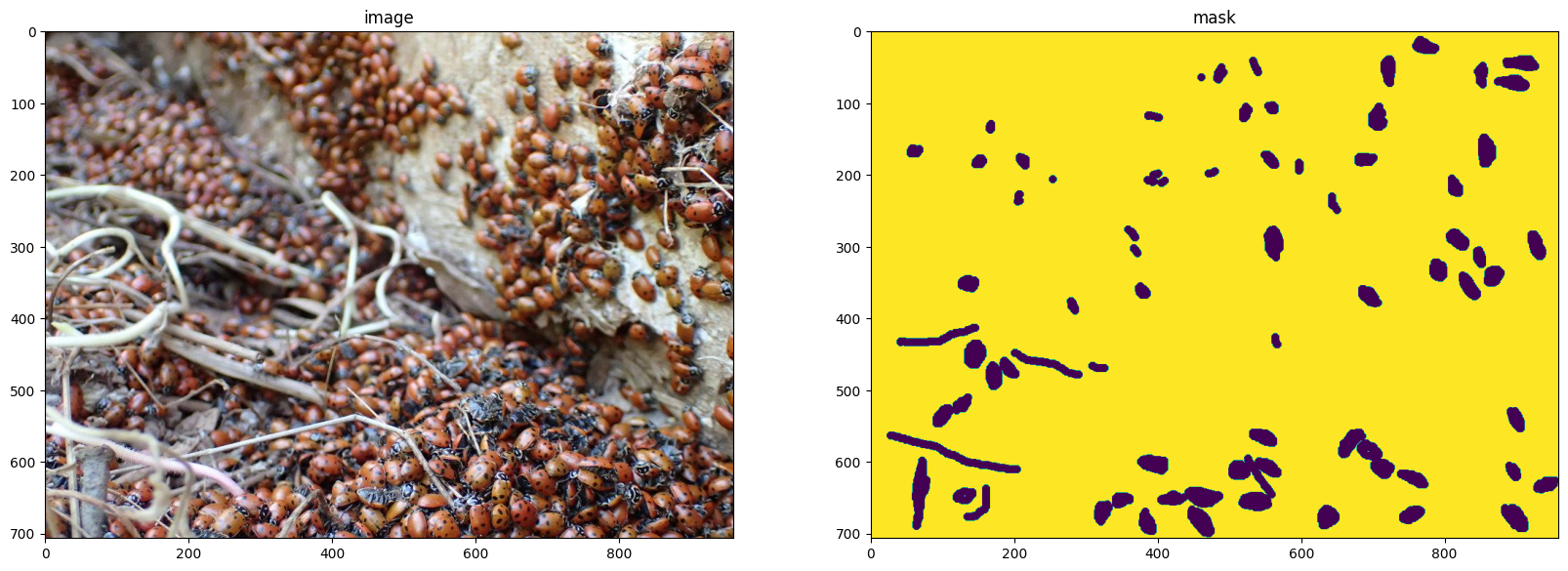
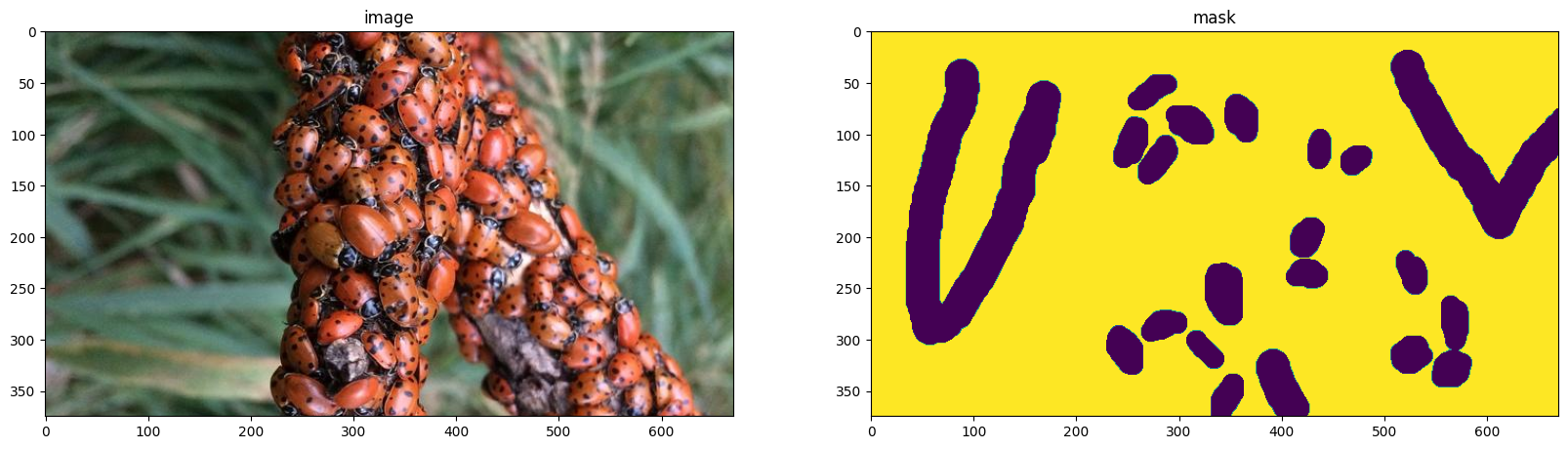